JSF h:link tag is used to render HTML anchor element.
JSF tag:
<h:link value="Click here" outcome="nextPage" /> |
<h:link value="Click here" outcome="nextPage" />
Rendered HTML tag:
<a href="/JavaServerFaces/faces/nextPage.xhtml">Click here</a> |
<a href="/JavaServerFaces/faces/nextPage.xhtml">Click here</a>
Note: We can add parameter to generated link by using f:param.
<h:link value="Click here" outcome="nextPage" >
<f:param name="id" value="1" />
</h:link> |
<h:link value="Click here" outcome="nextPage" >
<f:param name="id" value="1" />
</h:link>
Rendered HTML tag:
<a href="/JavaServerFaces/faces/nextPage.xhtml?id=1">Click here</a> |
<a href="/JavaServerFaces/faces/nextPage.xhtml?id=1">Click here</a>
Attributes of h:link tag.
Attribute |
Description |
id |
id for the tag |
binding |
Reference to the component used in a backing bean |
rendered |
A boolean value; false would suppress rendering |
styleClass |
Cascading stylesheet (CSS) class name |
value |
value binding |
valueChangeListener |
A method binding that responds to value changes |
converter |
Converter class name |
validator |
Class name of a validator attached to the component |
required |
A boolean; if true, marks the tag as required |
accesskey |
gives focus to an element |
accept |
Comma-separated list of content types for a form |
accept-charset |
Comma- or space-separated list of character encodings for a form. |
alt |
Alternative text for nontextual elements such as images |
border |
Pixel value for an element’s border width |
charset |
Character encoding for a linked resource |
coords |
Coordinates for an element whose shape is a rectangle, circle, or polygon |
dir |
Direction for text. Valid values are ltr (left to right) and rtl (right to left). |
hreflang |
Base language of a resource specified with the href attribute; |
lang |
Base language of an element’s attributes and text |
maxlength |
Maximum number of characters for text fields |
readonly |
Read-only state of an input field |
rel |
Relationship between the current page and linked page |
rev |
Reverse link from the anchor specified with href to the current document. |
style |
Inline style information |
tabindex |
Numerical value specifying a tab index |
target |
The name of a frame in which a document is opened |
title |
A title used for accessibility. Browsers typically create tooltips for the title’s value |
type |
Type of a link; for example, stylesheet |
width |
Width of an element |
onblur |
Event handler for losing focus |
onchange |
Event handler for value changes |
onclick |
Event handler for Mouse button clicked over the element |
ondblclick |
Event handler for Mouse button double-clicked |
onfocus |
Event handler for element received focus |
onkeydown |
Event handler for Key pressed |
onkeypress |
Event handler for Key pressed and released |
onkeyup |
Event handler for Key released |
onmousedown |
Event handler for Mouse button pressed |
onmousemove |
Event handler for mouse moved |
onmouseout |
Event handler for mouse left |
onmouseover |
Event handler for mouse moved onto |
onmouseup |
Event handler for mouse button released |
onreset |
Event handler for form reset |
onselect |
Event handler for Text selected |
Example:
test.xhtml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core"
xmlns:ui="http://java.sun.com/jsf/facelets">
<h:head>
<title>JSF link example.</title>
</h:head>
<h:body>
<h3>JSF link example.</h3>
<h:form>
<h:link value="Say Hello" outcome="welcome" />
</h:form>
</h:body>
</html> |
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core"
xmlns:ui="http://java.sun.com/jsf/facelets">
<h:head>
<title>JSF link example.</title>
</h:head>
<h:body>
<h3>JSF link example.</h3>
<h:form>
<h:link value="Say Hello" outcome="welcome" />
</h:form>
</h:body>
</html>
welcome.xhtml
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core"
xmlns:ui="http://java.sun.com/jsf/facelets">
<h:head>
<title>JSF link example.</title>
</h:head>
<h:body>
<h3>Hello World.</h3>
</h:body>
</html> |
<?xml version="1.0" encoding="UTF-8"?>
<!DOCTYPE html PUBLIC "-//W3C//DTD XHTML 1.0 Transitional//EN"
"http://www.w3.org/TR/xhtml1/DTD/xhtml1-transitional.dtd">
<html xmlns="http://www.w3.org/1999/xhtml"
xmlns:h="http://java.sun.com/jsf/html"
xmlns:f="http://java.sun.com/jsf/core"
xmlns:ui="http://java.sun.com/jsf/facelets">
<h:head>
<title>JSF link example.</title>
</h:head>
<h:body>
<h3>Hello World.</h3>
</h:body>
</html>
faces-config.xml
<?xml version="1.0" encoding="windows-1252"?>
<faces-config version="2.0"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xi="http://www.w3.org/2001/XInclude"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-facesconfig_2_0.xsd">
</faces-config> |
<?xml version="1.0" encoding="windows-1252"?>
<faces-config version="2.0"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xi="http://www.w3.org/2001/XInclude"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-facesconfig_2_0.xsd">
</faces-config>
web.xml
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="3.0"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd">
<servlet>
<servlet-name>faces</servlet-name>
<servlet-class>
javax.faces.webapp.FacesServlet
</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>faces</servlet-name>
<url-pattern>/faces/*</url-pattern>
</servlet-mapping>
</web-app> |
<?xml version="1.0" encoding="UTF-8"?>
<web-app version="3.0"
xmlns="http://java.sun.com/xml/ns/javaee"
xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance"
xsi:schemaLocation="http://java.sun.com/xml/ns/javaee
http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd">
<servlet>
<servlet-name>faces</servlet-name>
<servlet-class>
javax.faces.webapp.FacesServlet
</servlet-class>
</servlet>
<servlet-mapping>
<servlet-name>faces</servlet-name>
<url-pattern>/faces/*</url-pattern>
</servlet-mapping>
</web-app>
URL:
http://localhost:7001/JSFExample16/faces/test.xhtml
Output:
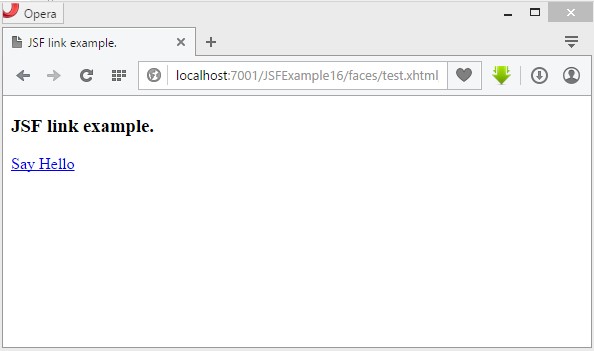
Click on Say Hello link.
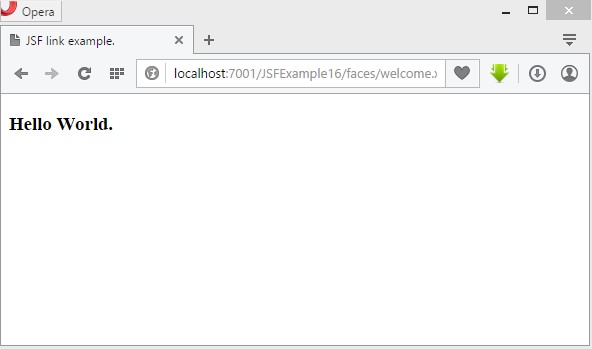
Download this example.